Easily Integrate
Sellsy's API using Konfig's TypeScript SDK
Growing API Companies trust Konfig's SDKs to onboard developers to their API.

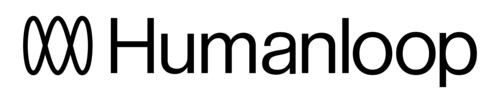
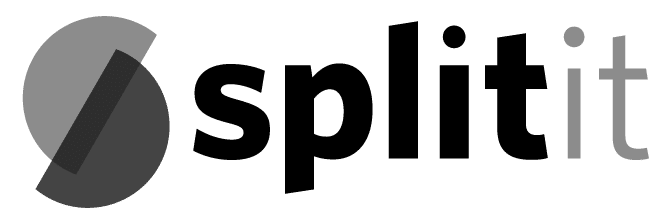
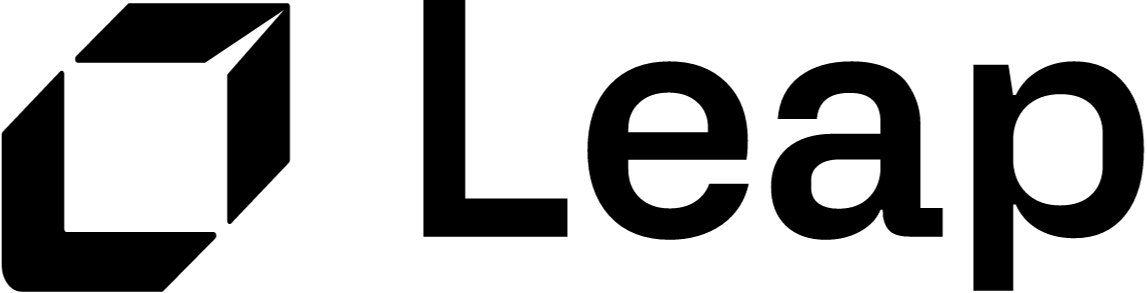

First, instantiate the SDK
It only takes a few lines of code.
Then, send your first request
We made it really easy.
Enjoy a buttery smooth developer experience with 351 SDK methods
Parameter
The order direction
The pagination limit
The pagination offset
Filters the fields returned in the response
Example:
- field[]=id
: Return the id
field
- field[]=address.id
: Return the id
field of the address
object
- field[]=addresses[].id
: Return the id
field of the address
objects
On endpoints that implement the embed
query parameter, if you specified embeds in your call, you will need to request the _embed
field as well.
Example:
- field[]=_embed
: Return all fields from all requested embeds
- field[]=_embed.address
: Return all fields from the address
embed
- field[]=_embed.company.name
: Return the name
field from the company
embed
Additional object included in the result.
Each embed object may require different oauth2 scopes than the main endpoint:
- company: companies.read
- individual: individuals.read
- owner: staffs.read
- contact: contacts.read
- related: by object type. Check the entire documentation to verify the usual scopes requested for the types returned
The order field
Response
Endpoint
Parameter
Additional object included in the result.
Each embed object may require different oauth2 scopes than the main endpoint:
- company: companies.read
- individual: individuals.read
- owner: staffs.read
- contact: contacts.read
- related: by object type. Check the entire documentation to verify the usual scopes requested for the types returned
Filters the fields returned in the response
Example:
- field[]=id
: Return the id
field
- field[]=address.id
: Return the id
field of the address
object
- field[]=addresses[].id
: Return the id
field of the address
objects
On endpoints that implement the embed
query parameter, if you specified embeds in your call, you will need to request the _embed
field as well.
Example:
- field[]=_embed
: Return all fields from all requested embeds
- field[]=_embed.address
: Return all fields from the address
embed
- field[]=_embed.company.name
: Return the name
field from the company
embed
Flag to trigger validation only (set to true to validate payload without persisting data)
Response
Contains all properties + _embed for an Item endpoint
Endpoint
No Parameters
Response
Comment deleted
Endpoint
Parameter
Additional object included in the result.
Each embed object may require different oauth2 scopes than the main endpoint:
- company: companies.read
- individual: individuals.read
- owner: staffs.read
- contact: contacts.read
- related: by object type. Check the entire documentation to verify the usual scopes requested for the types returned
Filters the fields returned in the response
Example:
- field[]=id
: Return the id
field
- field[]=address.id
: Return the id
field of the address
object
- field[]=addresses[].id
: Return the id
field of the address
objects
On endpoints that implement the embed
query parameter, if you specified embeds in your call, you will need to request the _embed
field as well.
Example:
- field[]=_embed
: Return all fields from all requested embeds
- field[]=_embed.address
: Return all fields from the address
embed
- field[]=_embed.company.name
: Return the name
field from the company
embed
Response
Contains all properties + _embed for an Item endpoint
Endpoint
Parameter
Additional object included in the result.
Each embed object may require different oauth2 scopes than the main endpoint:
- company: companies.read
- individual: individuals.read
- owner: staffs.read
- contact: contacts.read
- related: by object type. Check the entire documentation to verify the usual scopes requested for the types returned
Filters the fields returned in the response
Example:
- field[]=id
: Return the id
field
- field[]=address.id
: Return the id
field of the address
object
- field[]=addresses[].id
: Return the id
field of the address
objects
On endpoints that implement the embed
query parameter, if you specified embeds in your call, you will need to request the _embed
field as well.
Example:
- field[]=_embed
: Return all fields from all requested embeds
- field[]=_embed.address
: Return all fields from the address
embed
- field[]=_embed.company.name
: Return the name
field from the company
embed
Flag to trigger validation only (set to true to validate payload without persisting data)
Response
Contains all properties + _embed for an Item endpoint
Endpoint
Parameter
The order direction
The pagination limit
The pagination offset
Filters the fields returned in the response
Example:
- field[]=id
: Return the id
field
- field[]=address.id
: Return the id
field of the address
object
- field[]=addresses[].id
: Return the id
field of the address
objects
On endpoints that implement the embed
query parameter, if you specified embeds in your call, you will need to request the _embed
field as well.
Example:
- field[]=_embed
: Return all fields from all requested embeds
- field[]=_embed.address
: Return all fields from the address
embed
- field[]=_embed.company.name
: Return the name
field from the company
embed
Additional object included in the result.
Each embed object may require different oauth2 scopes than the main endpoint:
- company: companies.read
- individual: individuals.read
- owner: staffs.read
- contact: contacts.read
- related: by object type. Check the entire documentation to verify the usual scopes requested for the types returned
The order field
Response
Endpoint
How Konfig Works
Konfig collects APIs and automatically generates SDKs so you can focus on building your application.
Konfig maintains the highest quality collection of OpenAPI Specifications on the internet in a GitHub repository
We pull OpenAPI Specifications from public sources, fix any errors, and make sure they pass our lint rules. We continually make sure the repository is up-to-date and collect up-time and response time metrics for every API.
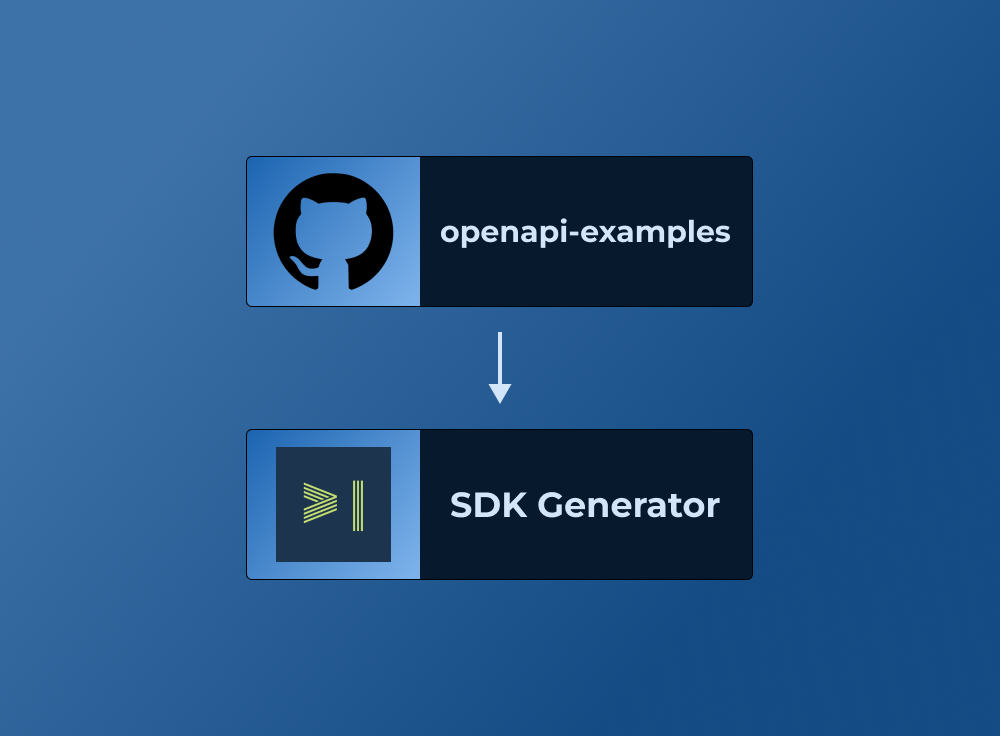
Generates AI-Augmented SDKs from openapi-examples
Our SDK generator is trusted by growing API companies and goes through a rigorous testing process to ensure the generated SDKs are high-quality and easy to use.
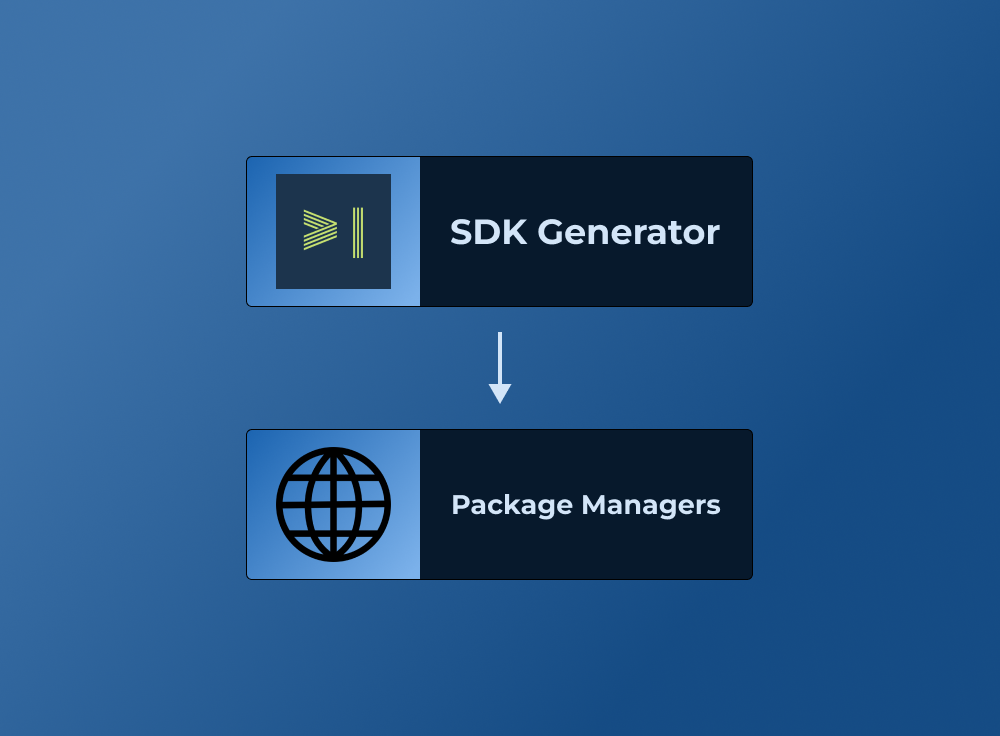
Publishes to standard package managers
We publish to standard package managers like npm, PyPI, and Maven so you can easily integrate the SDK into your application.
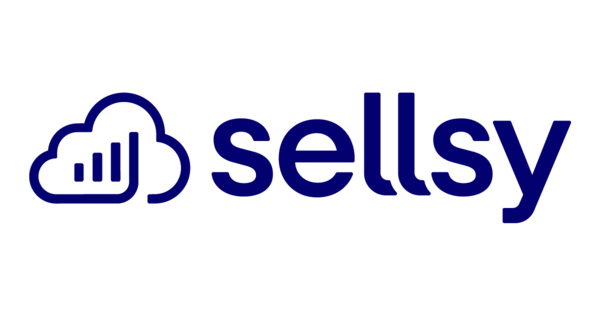
About Sellsy
Sellsy is a CRM, invoicing and pre-accounting suite for optimized business management and customer experience. A collaborative tool to give marketing, sales and administrative teams the means to be effective! 🚀 With 6,300 clients, Sellsy surrounds itself with almost 130 employees to carry out its projects. Sellsy remains, above all, a French company created by two entrepreneurs, Frédéric Coulais and Alain Mevellec, for entrepreneurs. Since its creation in 2009, Sellsy has placed innovation at the heart of its strategy, in order to offer companies simple, efficient and secure digital solutions. 👉 To learn more about Sellsy and its CRM suite, visit go.sellsy.com